What is Arduino and what is it for?
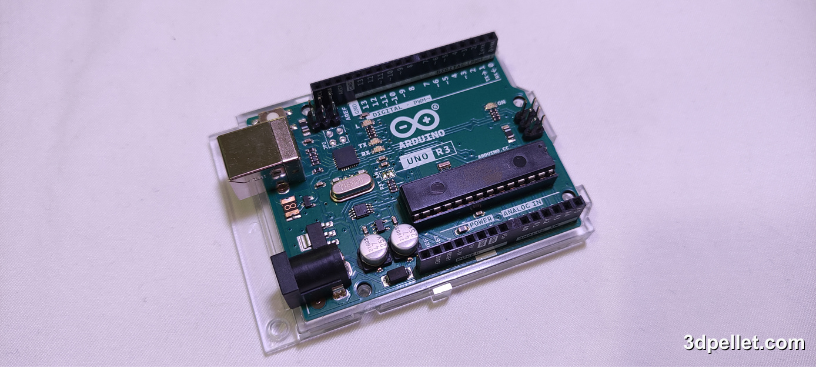
Arduino is both an open source electronics platform and a free software and hardware development company. The products sold by the company are distributed under the GPL and LGPL. Under these licenses Arduino allows the manufacture of Arduino boards and the distribution of the software by any individual. Although it should be noted that the word Arduino and the logos are a protected trademark.
Arduino boards are commercially available in the form of assembled boards or also in the form of kits. Most boards have an 8-bit Atmel microcontroller with the most common model being ATMEGA328P. Each microcontroller consists of various amounts of flash memory, pins, and functions. These can also be programmed to perform various tasks.
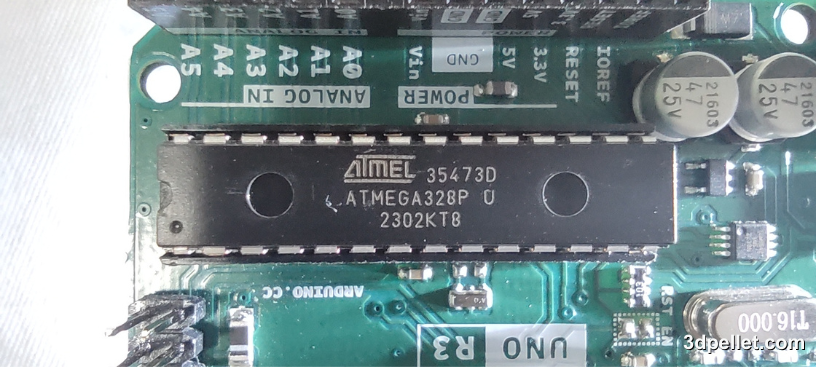
The boards use single or double row female pins that facilitate connections and incorporation into other circuits, such as sensors, motors, lights, etc. This versatility makes them ideal for IoT applications, wearables, 3D printing, and embedded environments.
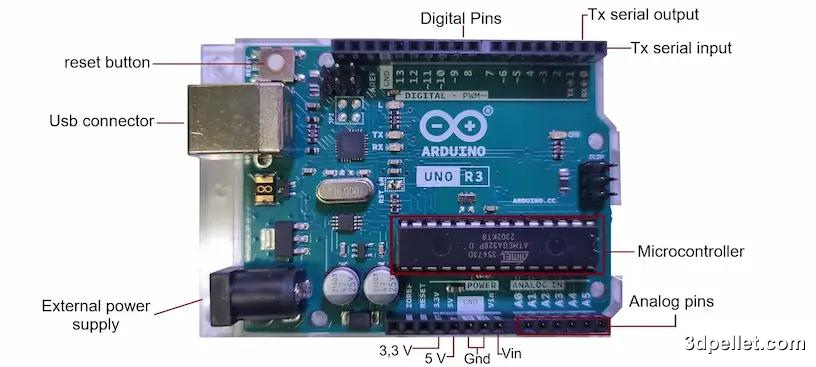
Some of its fundamental parts are the following:
-
Microcontroller: This is the brain of an Arduino and is the component into which we load the programs. Think of it as a small computer, designed to run only a specific number of things.
-
USB Port: Used to connect your Arduino board to a computer.
-
Digital Pins: Pins that use digital logic (LOW/HIGH). Commonly used for switches and to turn an LED on/off.
-
Analog pins: These pins can read analog values with a resolution of 10 to 16 bits depending on the board. analogRead()
-
5V and 3.3V pins: These pins are used to power external components with 5V or 3.3V respectively.
-
GND: Used to complete a circuit, it is also known as common or ground.
-
VIN: It is a voltage input, where an external power supply can be connected.
Depending on the Arduino board you will find many more components. The items listed above are usually found on any Arduino board.
Arduino IDE
To program the microcontroller on Arduino hardware, it is possible to use several compilers and programming languages. One of the most used is the Arduino integrated development environment (IDE). This is a cross-platform application (for Windows, macOS, Linux) that is written in Java.
The Arduino IDE supports the C and C++ languages using special code structuring rules. User-written code only requires two basic functions. One to start the sketch called setup() and another for the main cycle of the program called loop().
A program that can be run on our board without having to configure anything is the one that makes the LED on the board blink. Most Arduino boards contain an LED and a current limiting resistor connected between pin 13. This allows us to turn this LED on or off by configuring this pin 13 as an output. In the following code we see how to configure pin 13 as an output within the setup() function and then in the loop() function we turn it on by placing a high voltage on this pin with the digitalWrite() function followed by a delay and then turning off the LED by applying a low voltage to it. This will repeat indefinitely as long as we have the Arduino powered, causing the LED on the board to turn on and off periodically.
1const int LED_PIN = 13; // LED pin number
2
3void setup() {
4 pinMode(LED_PIN, OUTPUT); // Pin 13 is configured as a digital output.
5}
6
7void loop() {
8 digitalWrite(LED_PIN, HIGH); // We turn on the LED.
9 delay(1000); // We wait 1 second (1000 milliseconds).
10 digitalWrite(LED_PIN, LOW); // We turn off the LED.
11 delay(1000); // We wait 1 second
12}
Arduino Key Features
As a summary, the Arduino platform can be described using the following key characteristics:
-
Hardware and Open Source: Arduino hardware and software are open source, meaning that the design and source code are available for anyone to study, modify and share.
-
Development Environment (IDE): Arduino provides an easy-to-use development environment that simplifies writing, uploading and debugging code on Arduino boards.
-
Versatility: It can be used for a wide variety of projects, from simple experiments to more complex and interactive systems.
-
Active Community: Arduino has a large community of users and developers who share knowledge, projects and collaborate in the development of new ideas.
-
Wide Variety of Boards: There are different models of Arduino boards, each with its own characteristics and capabilities. Some of the well-known boards include Arduino Uno, Arduino Mega, Arduino Nano, and many more.
-
Extensibility: You can add modules and shields (expansion boards) to add specific functionalities to your project without having to design everything from scratch.
Arduino has become a popular tool for enthusiasts, students, and professionals who want to explore electronics and programming in an accessible way. Additionally, the simplicity and flexibility of Arduino make it ideal for educational projects, prototyping, and product development.